Nối tiếp Bài tập Python cơ bản 2020 – Phần 1 (Đề bài), chúng mình gửi tới bạn bộ lời giải mang tính tham khảo cho những bài toán trên. Bạn cũng đừng ngần ngại chia sẻ tới Got It những giải pháp thú vị khác nhé! Happy Coding!
I. Bài tập Python mức độ 1
1. Character Input
- Đề bài: Tạo một chương trình yêu cầu người dùng nhập tên và tuổi của họ. Gửi lại họ một tin nhắn cho biết năm họ sẽ tròn 100 tuổi.
- Lời giải:
name = input("What is your name: ")
age = int(input("How old are you: "))
year = str((2020 - age) + 100)
print(name + " will be 100 years old in the year " + year)
2. List Ends
- Đề bài: Viết chương trình lấy một list các con số (Ví dụ: a = [2, 4, 6, 8, 10]) và tạo một list mới chỉ gồm các phần tử đầu tiên và cuối cùng của list đã cho. Lưu ý: Viết code này bên trong một hàm.
- Lời giải:
def list_ends(arr):
return [arr[0], arr[-1]]
3. Birthday Dictionaries
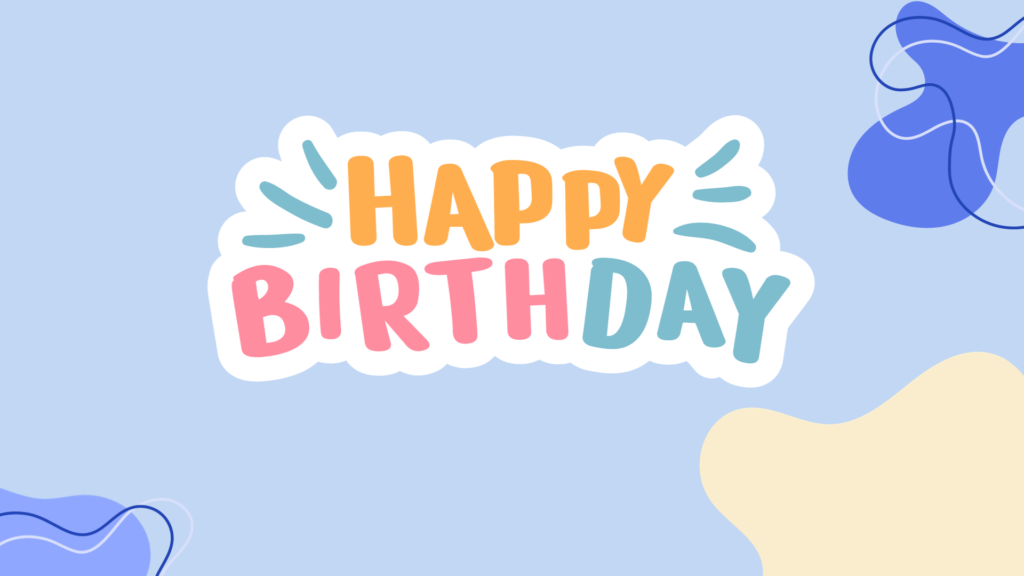
- Đề bài: Đây là một bài tập giúp chúng ta theo dõi ngày sinh của bạn mình và có thể tìm thấy thông tin đó dựa trên tên của họ. Hãy tạo một Dictionary (Bộ từ điển) gồm tên và ngày sinh trong file của bạn. Khi chương trình chạy, nó sẽ yêu cầu người dùng nhập tên và trả lại đúng ngày sinh của người đó cho họ. Tương tác có thể được hình dung như sau:
>>> Welcome to the birthday dictionary. We know the birthdays of:
Albert Einstein
Bill Gates
Steve Jobs
>>> Who's birthday do you want to look up?
Bill Gates
>>> Bill Gates's birthday is 28/10/1955
- Lời giải:
birthdays = {
'Albert Einstein': '03/14/1879',
'Benjamin Franklin': '01/17/1706',
'Ada Lovelace': '12/10/1815',
'Donald Trump': '06/14/1946',
'Rowan Atkinson': '01/6/1955'
}print('Welcome to the birthday dictionary. We know the birthdays of:')
for name in birthdays:
print(name)print('Whose birthday do you want to look up?')
name = input()
if name in birthdays:
print(f'{name}\'s birthday is {birthdays[name]}')
else:
print(f'Sadly, we don\'t have {name}\'s birthday.')
4. Element Search
- Đề bài: Viết một hàm nhận một list các số có sắp xếp thứ tự từ nhỏ đến lớn và một số khác. Hàm đó sẽ xác định xem số đã cho có nằm trong list hay không và trả về, in ra một Boolean thích hợp. Yêu cầu sử dụng Binary Search (tìm kiếm nhị phân).
- Lời giải:
def iterative_binary_search(ordered_list: list, number):
"""Implement binary search with while loop"""
left = 0
right = len(ordered_list) - 1
while left <= right:
middle = left + (right - left) // 2
if ordered_list[middle] == number:
return True
elif number < ordered_list[middle]:
right = middle - 1
else:
left = middle + 1
return False
def recursive_binary_search(ordered_list: list, number):
"""
Implement binary search recursively
Concepts:
- Recursion
- List indexing and slicing
Downside:
- Using list slicing costs more memory
"""
if len(ordered_list) == 0:
return False
if len(ordered_list) == 1:
return ordered_list[0] == number
if number < ordered_list[0] or number > ordered_list[-1]:
return False
middle = len(ordered_list) // 2
if ordered_list[middle] == number:
return True
elif number < ordered_list[middle]:
return binary_search_recursive(ordered_list[:middle], number)
else:
return binary_search_recursive(ordered_list[middle + 1:], number)
II. Bài tập Python mức độ 2
1. Divisors
- Đề bài: Tạo một chương trình hỏi người dùng một con số và in ra tất cả ước số của con số đó.
- Lời giải:
try:
number = int(input("Please choose a number to divide: "))
except ValueError:
print("We only accept integers.")
exit(0)
if number == 0:
print("All non-zero integers are divisors of 0")
exit(0)
# Accept both negative and positive number
# Disivors can be negative or postive as well
divisors = []
for i in range(1, abs(number) + 1):
if number % i == 0:
divisors.extend([i, -1 * i])
print(divisors)
2. String Lists
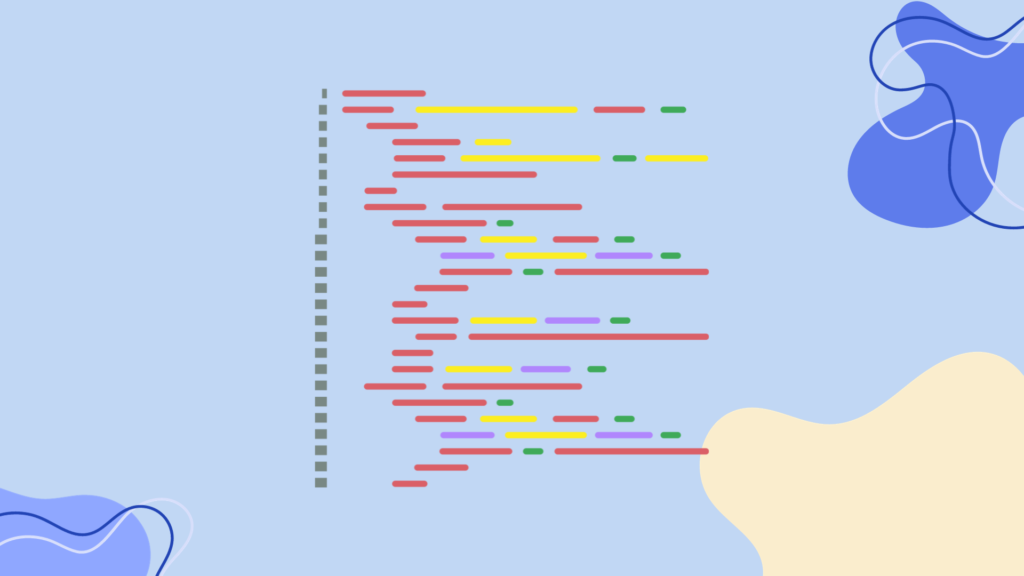
- Đề bài: Yêu cầu người dùng cung cấp một chuỗi và cho biết đó có phải một palindrome không (palindrome là một chuỗi có thể được viết xuôi hay viết ngược vẫn chỉ cho ra chính nó).
- Lời giải:
def is_palindrome(string) -> bool:
for i in range(0, int(len(string) / 2)):
if string[i] != str[len(string) - i - 1]:
return False
return True
def check_palindrome(string: str) -> bool:
# Use built-in function to reverse a string then compare with original string
return string == string[::-1]
if __name__ == "__main__":
input_string = input("Please enter a string: ")
print(is_palindrome(input_string))
3. List Less Than Ten
- Đề bài: Lấy một list, ví dụ như sau:
a = [1, 1, 2, 3, 5, 9, 12, 23, 35, 56, 88]
Viết một chương trình in ra tất cả các phần tử có giá trị nhỏ hơn 5. Ngoài ra, bạn có thể làm thêm các yêu cầu sau:
- Thay vì in từng phần tử một, hãy in ra một list mới có tất cả các phần tử nhỏ hơn 5 từ list
a
ban đầu. - Khi hỏi thêm người dùng một con số khác (số X), chương trình có thể trả lại một list mới có chứa các phần tử nhỏ hơn X từ list
a
ban đầu.
- Lời giải:
def lessThanTen(numbers, givenNumber):
newList = [];
for number in numbers:
if (number < givenNumber):
newList.append(number)
return newList
if __name__ == "__main__":
list = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
givenNumber = input("Type an integer:")
print(lessThanTen(list, givenNumber))
4. List Overlap Comprehensions
- Đề bài: Lấy hai lists, ví dụ như sau:
a = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
b = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13]
Viết chương trình cho ra một list chỉ chứa những phần tử chung giữa các list đã cho (không được trùng nhau). Đảm bảo rằng chương trình có thể hoạt động trên hai lists có kích thước khác nhau. Bạn cần sử dụng ít nhất một List Comprehension (List Comprehension là cách viết code ngắn gọn để tạo một danh sách phức tạp).
- Lời giải:
import random
a = random.sample(range(1,30), 12)
b = random.sample(range(1,30), 16)
result = [i for i in set(a) if i in b]
5. Fibonacci
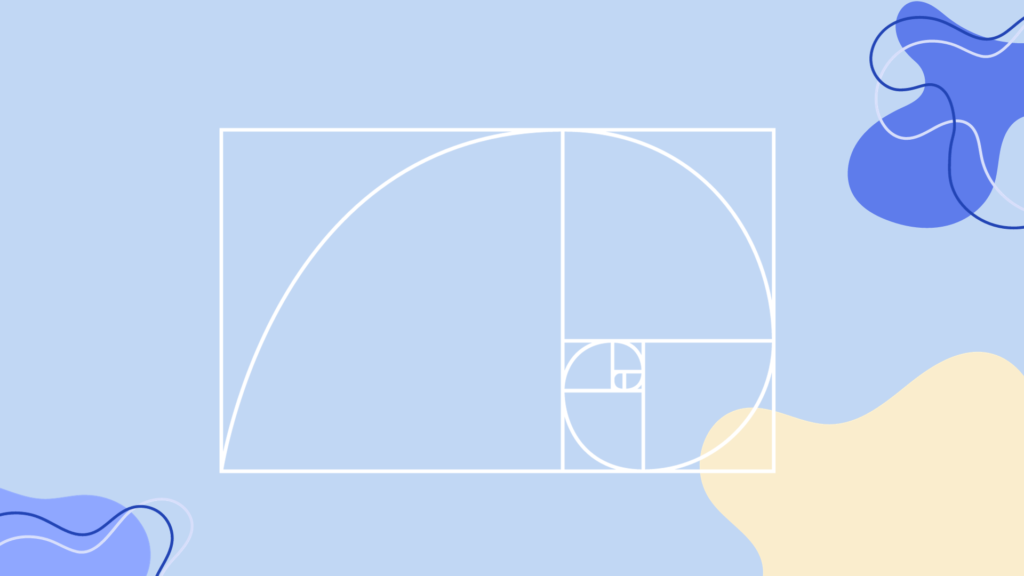
- Đề bài: Viết chương trình hỏi người dùng cần tạo bao nhiêu số trong dãy Fibonacci và tạo chúng. Chuỗi Fibonacci là một dãy số trong đó số tiếp theo trong dãy là tổng của hai số trước đó. Ví dụ của một chuỗi Fibonacci như sau: 1, 1, 2, 3, 5, 8, 13,…
- Lời giải:
def fibonacci(): num = int(input("How many numbers that generates?: ")) i = 1
if num < 0:
print("Please enter a non-negative number.")
exit(0) elif num == 0: fib = [] elif num == 1: fib = [1] elif num == 2: fib = [1,1] else: fib = [1,1] while i < (num - 1): fib.append(fib[i] + fib[i-1]) i += 1 return fib
print(fibonacci()) input()
6. List Remove Duplicates
- Đề bài: Viết một hàm để nhận một list và trả lại một list mới loại bỏ mọi phần tử bị trùng nhau trong list ban đầu. Trong đó, viết hai loại hàm: Một sử dụng vòng lặp (Loop), một sử dụng Set trong Python.
- Lời giải:
def remove_duplicates_using_loops(elements: list) -> list:
result: list = []
for element in elements:
if element not in result:
result.append(element)
return result
def remove_duplicates_using_set(elements: list) -> list:
return list(set(elements))
# Try our functions
test_list = [1, 2, 3, 4, 3, 2, 1]
print('Result using loops: ', remove_duplicates_using_loops(test_list))
print('Result using set: ', remove_duplicates_using_set(test_list))
# Output:
# Result using loops: [1, 2, 3, 4]
# Result using set: [1, 2, 3, 4]
III. Bài tập Python mức độ 3
1. Rock Paper Scissors
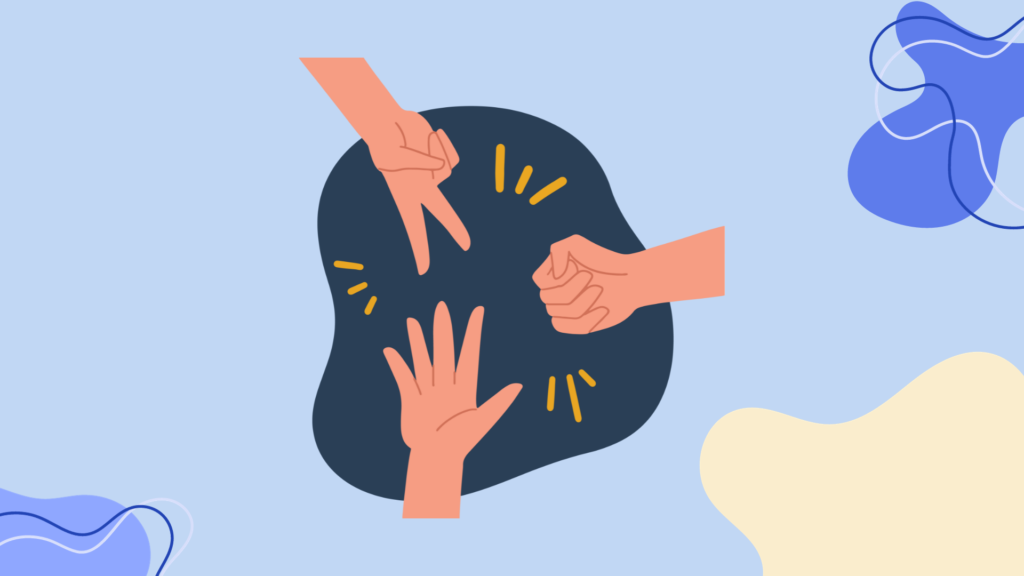
- Đề bài: Tạo game Đấm – Lá – Kéo dành cho hai người chơi. Trong đó, chương trình sẽ yêu câu người dùng nhập lượt chơi, so sánh kết quả, gửi tin nhắn chúc mừng tới người thắng cuộc và hỏi họ có muốn bắt đầu chơi lại một game mới không.
- Lời giải:
import sys
user1 = input("What's your name?")
user2 = input("And your name?")
user1_answer = input("%s, do yo want to choose rock, paper or scissors?" % user1)
user2_answer = input("%s, do you want to choose rock, paper or scissors?" % user2)
def compare(u1, u2):
if u1 == u2:
return("It's a tie!")
elif u1 == 'rock':
if u2 == 'scissors':
return("Rock wins!")
else:
return("Paper wins!")
elif u1 == 'scissors':
if u2 == 'paper':
return("Scissors win!")
else:
return("Rock wins!")
elif u1 == 'paper':
if u2 == 'rock':
return("Paper wins!")
else:
return("Scissors win!")
else:
return("Invalid input! You have not entered rock, paper or scissors, try again.")
sys.exit()
print(compare(user1_answer, user2_answer))
2. Check Primality Functions
- Đề bài: Yêu cầu người dùng nhập một số và xác định xem đó có phải là số nguyên tố hay không. Bạn có thể sử dụng kết quả từ bài tập Divisors (phần I) để giúp mình làm tiếp bài này.
- Lời giải:
def check_prime_using_loops_optimized(n: int) -> bool:
"""
Check prime using for loops using a more optimal way that checks from i = 2 -> sqrt(n) + 1
Time complexity: O(sqrt(n))
"""
import math
if n > 1:
for i in range(2, int(math.sqrt(n) + 1)):
if n % i == 0:
return False
return True
else:
return False
def check_prime_using_list_comprehensions(n: int) -> bool:
"""
Check prime using list comprehensions
Time complexity: O(n)
"""
divisors = [x for x in range(2, n) if n % x == 0]
return len(divisors) == 0
3. Reverse Word Order
- Đề bài: Viết một chương trình (sử dụng các hàm) yêu cầu người dùng cung cấp một chuỗi dài chứa nhiều từ. In lại cho người dùng một chuỗi mới với thứ tự từ ngữ được đảo ngược lại với list ban đầu. Ví dụ, khi người dùng nhập chuỗi:
My name is Got It-ian
thì họ sẽ nhận lại được một kết quả như sau:
Got It-ian is name My
- Lời giải:
def reverse_word(string):
return ' '.join(string.split()[::-1])
4. Cows and Bulls
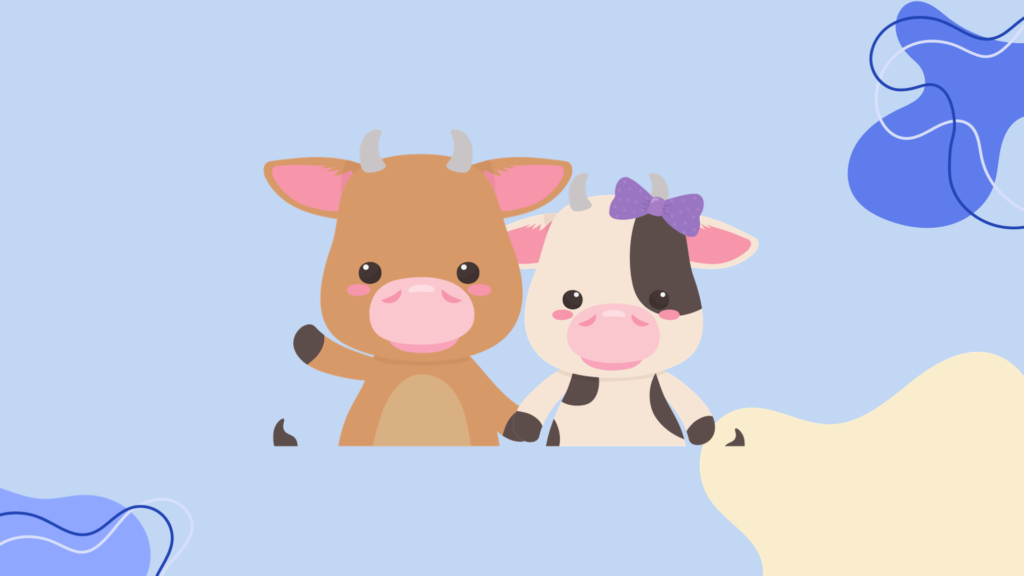
- Đề bài: Tạo trò chơi “Cows and Bulls” với cách thức hoạt động như sau:
- Tạo ngẫu nhiên một con số có 4 chữ số. Yêu cầu người chơi đoán con số đó.
- Khi người chơi đoán đúng một chữ số nào đó ở đúng vị trí, họ sẽ có một “Cow”. Với mỗi chữ số sai, họ sẽ có một “Bull”.
- Mỗi khi người dùng đưa ra phỏng đoán, hãy cho họ biết họ có bao nhiêu “Cows” và “Bulls”. Khi người dùng đoán đúng số, trò chơi kết thúc. Theo dõi số lần đoán mà người dùng thực hiện trong suốt trò chơi và họ biết khi kết thúc.
Giả sử, máy tính tạo ra một con số là 1038. Một tương tác sẽ diễn ra như sau:
Welcome to the Cows and Bulls Game!
Enter a number:
>>> 1234
2 cows, 0 bulls
>>> 1256
1 cow, 1 bull
...
- Lời giải:
import random
def compare_numbers(number, user_guess):
cow = 0
bull = 0
for i in range(len(number)):
if user_guess[i] == number[i]:
cow += 1
else:
if user_guess[i] in number:
bull += 1
return cow, bull
if __name__ == "__main__":
playing = True # gotta play the game
number = str(random.randint(1000, 9999)) # random 4 digit number
guesses = 0
print("Let's play a game of Cowbull!")
print("For every correct digit in the right place, you get a cow. For every correct digit in the wrong place, you get a bull.")
print("The game ends when you get 4 cows!")
print("Type exit at any prompt to exit.")
while playing:
user_guess = input("Give me your best guess: ")
if user_guess == "exit":
print("Exited the game.")
break
if len(user_guess) != 4:
print("Invalid answer, please try again!")
continue
cow, bull = compare_numbers(number, user_guess)
guesses += 1
print(f"You have {str(cow)} cows, and {str(bull)} bulls.")
if user_guess == number:
playing = False
print(f"You win the game after {guesses} guesses! The number was {number}.")
break
else:
print("Your guess isn't quite right, try again.\n")
5. Password Generator
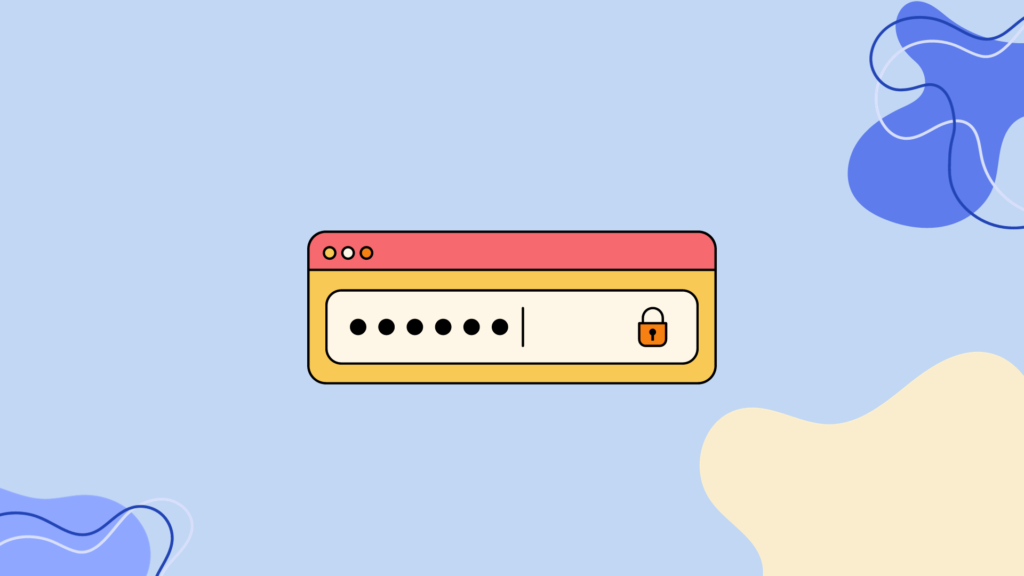
- Đề bài: Viết trình tạo mật khẩu ngẫu nhiên bằng Python. Bạn có thể tuỳ ý sáng tạo nhưng một mật khẩu mạnh được gợi ý là có sự kết hợp của chữ thường, chữ hoa, số và ký hiệu. Chương trình cần tạo một mật khẩu mới mỗi khi người dùng yêu cầu reset password.
- Lời giải:
import random
def generate_secure_password(length=16, numbers=True, uppercase=True, lowercase=True, symbols=True):
# For security reason, we shouldn't allow users to generate password with length less than 8
if length < 8:
raise Exception("Your password length should not be less than 8.")
if all([lowercase is False, uppercase is False, numbers is False, symbols is False]):
raise Exception("Your password should include at least lowercase, uppercase, numbers or symbols characters.")
# Define groups of characters that can be included in the password
password_groups = [
(uppercase, 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'),
(lowercase, 'abcdefghijklmnopqrstuvwxyz'),
(numbers, '0123456789'),
(symbols, '!"#$%&\'()*+,-./:;<=>?@^[\\]^_`{|}~'),
]
# Filter out unwanted types of character in the password
included_password_groups = list(filter(lambda password_group: password_group[0], password_groups))
# Generate the password
password_characters = []
for i in range(length):
password_group_index = i % len(included_password_groups)
_, group_characters = included_password_groups[password_group_index]
password_characters.append(random.choice(group_characters))
random.shuffle(password_characters)
return ''.join(password_characters)
[…] Đọc tiếp: Tổng hợp bài tập Python cơ bản 2020 – Phần 2 (Lời giải) […]